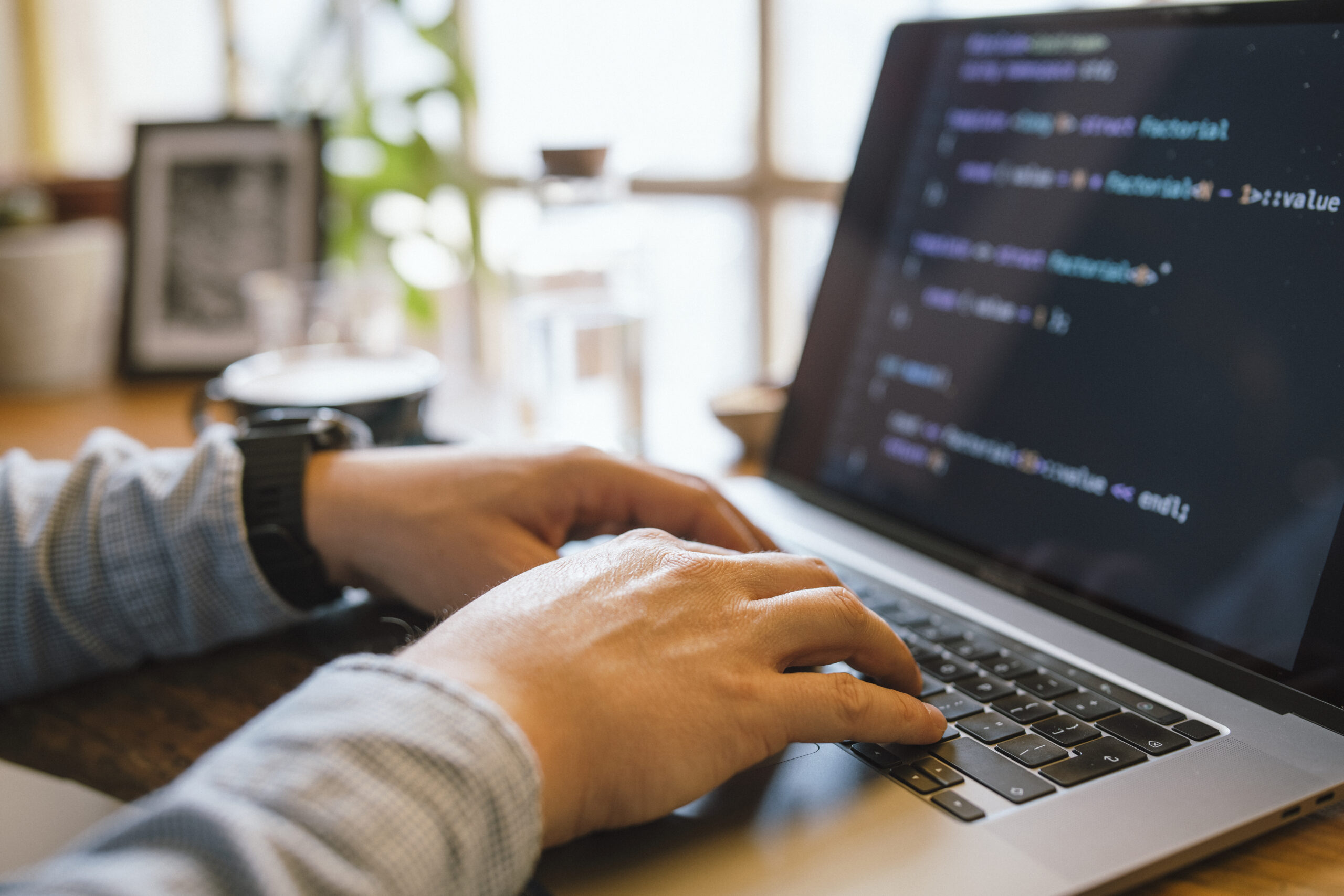
Debugging is Probably the most essential — nevertheless typically forgotten — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Imagine methodically to unravel issues effectively. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve several hours of irritation and dramatically improve your productivity. Listed below are numerous procedures to help builders level up their debugging game by me, Gustavo Woltmann.
Learn Your Tools
One of several fastest strategies builders can elevate their debugging techniques is by mastering the instruments they use everyday. Although composing code is a person Component of progress, figuring out tips on how to interact with it effectively all through execution is equally crucial. Contemporary development environments come Geared up with strong debugging capabilities — but lots of builders only scratch the surface of what these applications can perform.
Just take, for instance, an Built-in Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, step by way of code line by line, as well as modify code around the fly. When made use of accurately, they let you notice precisely how your code behaves all through execution, which can be invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They assist you to inspect the DOM, check community requests, see true-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and community tabs can turn annoying UI issues into manageable jobs.
For backend or procedure-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Manage in excess of functioning processes and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, come to be comfortable with Edition Management devices like Git to understand code background, obtain the precise moment bugs were introduced, and isolate problematic alterations.
In the long run, mastering your applications implies heading over and above default options and shortcuts — it’s about acquiring an personal knowledge of your improvement setting to make sure that when issues arise, you’re not lost at midnight. The better you recognize your instruments, the greater time it is possible to shell out fixing the actual difficulty rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — steps in effective debugging is reproducing the condition. Right before leaping in the code or generating guesses, developers need to have to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, repairing a bug gets to be a game of prospect, generally resulting in squandered time and fragile code improvements.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire questions like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you've, the a lot easier it gets to isolate the exact ailments below which the bug takes place.
As soon as you’ve collected enough data, attempt to recreate the condition in your local natural environment. This could necessarily mean inputting the exact same info, simulating identical user interactions, or mimicking process states. If the issue seems intermittently, consider crafting automated assessments that replicate the edge situations or point out transitions concerned. These assessments not merely assistance expose the trouble and also prevent regressions Sooner or later.
In some cases, the issue could possibly be surroundings-precise — it'd take place only on selected functioning methods, browsers, or beneath specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t simply a move — it’s a state of mind. It calls for tolerance, observation, in addition to a methodical method. But after you can regularly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, you can use your debugging tools far more proficiently, exam opportunity fixes properly, and connect extra Evidently along with your crew or consumers. It turns an abstract complaint into a concrete challenge — Which’s where developers thrive.
Study and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Improper. As opposed to seeing them as irritating interruptions, builders should really study to deal with error messages as direct communications from your method. They often show you just what exactly took place, in which it happened, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message diligently As well as in complete. Several developers, specially when beneath time stress, look at the primary line and instantly start making assumptions. But further within the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into search engines — examine and recognize them first.
Split the error down into areas. Is it a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line number? What module or operate brought on it? These inquiries can guidebook your investigation and issue you towards the liable code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, As well as in Those people situations, it’s very important to examine the context through which the mistake occurred. Examine related log entries, input values, and recent variations in the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger challenges and provide hints about possible bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems speedier, reduce debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is one of the most potent instruments inside of a developer’s debugging toolkit. When utilized successfully, it provides true-time insights into how an software behaves, serving to you understand what’s happening beneath the hood while not having to pause execution or step from the code line by line.
A good logging method begins with being aware of what to log and at what stage. Widespread logging amounts incorporate DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info throughout growth, Data for standard occasions (like profitable start off-ups), WARN for possible problems that don’t break the appliance, ERROR for actual problems, and Lethal if the process can’t keep on.
Prevent flooding your logs with extreme or irrelevant knowledge. Excessive logging can obscure crucial messages and slow down your program. Concentrate on key situations, condition adjustments, enter/output values, and demanding determination points in the code.
Structure your log messages Plainly and persistently. Contain context, such as timestamps, request IDs, and performance names, so it’s easier to trace issues in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to keep track of how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without halting This system. They’re especially precious in manufacturing environments where stepping by code isn’t feasible.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, intelligent logging is about stability and clarity. Which has a nicely-considered-out logging technique, you can decrease the time it takes to spot difficulties, gain further visibility into your purposes, and improve the All round maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a type of investigation. To properly determine and correct bugs, builders must technique the method similar to a detective resolving a mystery. This attitude will help break down complicated concerns into workable sections and abide by clues logically to uncover the root result in.
Start out by accumulating proof. Consider the indicators of the condition: error messages, incorrect output, or efficiency concerns. The same as a detective surveys a criminal offense scene, accumulate just as much suitable details as it is possible to with no leaping to conclusions. Use logs, examination situations, and consumer stories to piece jointly a clear image of what’s occurring.
Upcoming, sort hypotheses. Check with on your own: What may very well be resulting in this habits? Have any alterations not too long ago been created towards the codebase? Has this situation transpired prior to under identical instances? The target is usually to narrow down possibilities and detect potential culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled environment. When you suspect a certain perform or ingredient, isolate it and confirm if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results guide you closer to the reality.
Pay out close awareness to smaller specifics. Bugs often cover inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or simply a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally being familiar with it. Short term fixes may perhaps conceal the actual dilemma, only for it to resurface afterwards.
Finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for potential challenges and support others recognize your reasoning.
By thinking just like a detective, builders can sharpen their analytical skills, strategy complications methodically, and turn out to be simpler at uncovering hidden concerns in advanced systems.
Publish Assessments
Crafting tests is one of the best solutions to improve your debugging capabilities and In general advancement performance. Tests not just support capture bugs early but will also function a security net that gives you assurance when creating adjustments to the codebase. A very well-analyzed software is here much easier to debug mainly because it helps you to pinpoint exactly exactly where and when an issue takes place.
Begin with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a selected bit of logic is Doing the job as envisioned. Any time a take a look at fails, you promptly know wherever to seem, drastically minimizing time spent debugging. Device tests are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Future, combine integration exams and finish-to-end tests into your workflow. These help make sure several areas of your application get the job done collectively easily. They’re significantly handy for catching bugs that take place in complex units with various parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically regarding your code. To test a element effectively, you need to grasp its inputs, expected outputs, and edge scenarios. This level of knowledge Normally sales opportunities to better code framework and fewer bugs.
When debugging a concern, creating a failing take a look at that reproduces the bug can be a strong starting point. After the take a look at fails consistently, it is possible to focus on repairing the bug and enjoy your check go when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—aiding you capture extra bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Option just after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you simply wrote just hours earlier. During this state, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Numerous developers report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to maneuver all over, stretch, or do something unrelated to code. It could really feel counterintuitive, Primarily below limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a wise strategy. It provides your Mind space to breathe, enhances your standpoint, and assists you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Just about every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can instruct you something useful in case you take some time to replicate and review what went wrong.
Begin by asking oneself a number of critical thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater tactics like device tests, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, several of the very best builders aren't those who write best code, but those that repeatedly discover from their problems.
In the end, Every single bug you repair provides a new layer to the skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, follow, and tolerance — but the payoff is big. It would make you a far more effective, assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.